Threads in Java ๐งถ
3 min read
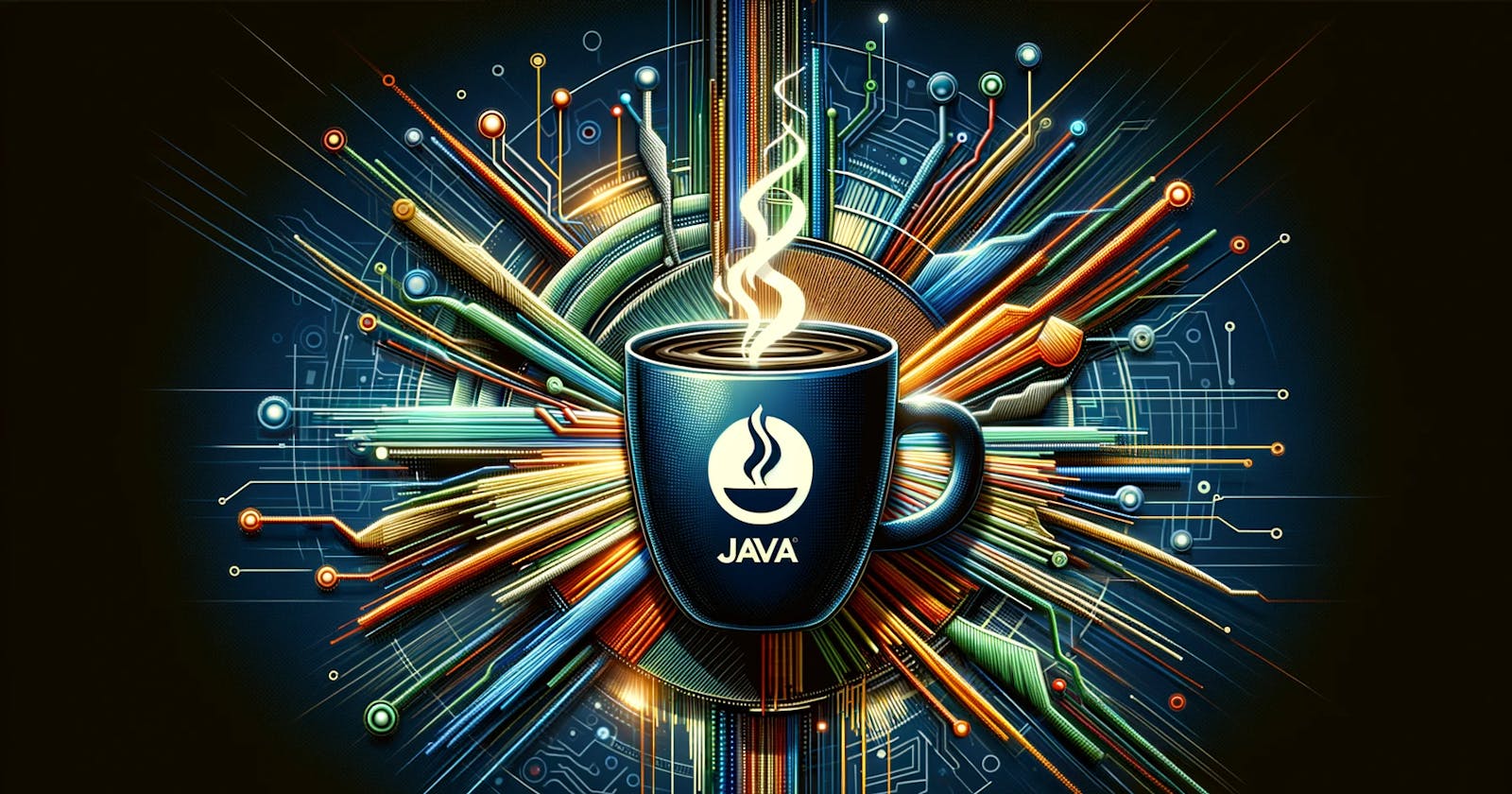
What are Threads in Java? ๐งต (Simple Explanation)
Imagine you're in a kitchen ๐ณ cooking a meal. You're the chef (the main program), and you have several tasks like chopping vegetables ๐ฅ, boiling water ๐ง, and frying eggs ๐ณ. In a normal scenario (single-threaded), you'd do each task one after the other. But what if you could have helpers (threads) who could do some of these tasks at the same time? That's exactly what threads in Java do!
In Java, a thread is like a helper ๐ฅ in your program. It can run parts of your code in parallel with other parts. This means you can do multiple things at the same time, like playing music ๐ต while downloading a file ๐พ.
How to Create Threads ๐
There are mainly two ways to create a thread in Java:
By extending the
Thread
class:It's like writing a recipe ๐ for your helper.
You write a class that extends
Thread
and put the instructions in therun()
method.
By implementing the
Runnable
interface:This is like giving a list of tasks ๐ to an existing helper.
You write a class that implements
Runnable
and put the tasks in therun()
method. Then you give this list to aThread
object.
A Simple Example ๐
Let's say you're writing a program that sends an email โ๏ธ and at the same time calculates the sum of numbers ๐ข.
Implementing Runnable
Interface
// Task 1: Sending an Email
class EmailSender implements Runnable {
public void run() {
// Code to send an email
System.out.println("Email sent! ๐ง");
}
}
// Task 2: Calculate Sum
class SumCalculator implements Runnable {
public void run() {
int sum = 0;
for (int i = 1; i <= 10; i++) {
sum += i;
}
System.out.println("Sum is: " + sum + " ๐งฎ");
}
}
public class Main {
public static void main(String[] args) {
// Create tasks
EmailSender emailSender = new EmailSender();
SumCalculator sumCalculator = new SumCalculator();
// Create threads
Thread thread1 = new Thread(emailSender);
Thread thread2 = new Thread(sumCalculator);
// Start threads
thread1.start();
thread2.start();
}
}
In this example, EmailSender
and SumCalculator
are like two separate helpers ๐ฅ๐ฅ. The Main
class creates these helpers and starts them. They run simultaneously, so the email can be sent ๐ while the sum is being calculated ๐ง .
Benefits of Using Threads ๐
Efficiency: Your program can do multiple things at once, like a chef who can cook and talk on the phone simultaneously ๐.
Better Use of Resources: If you have to wait for something (like waiting for a file to download), you can do other tasks in the meantime โณ.
Things to Keep in Mind โ ๏ธ
Thread Safety: When threads share resources (like ingredients in a kitchen), you need to be careful. If not handled properly, it can lead to problems (like two helpers using the same frying pan at the same time) ๐ณ.
Complexity: Using threads can make your program more complex, like managing a kitchen with many helpers. You need to coordinate them well ๐คน.