π Passing Inputs to Tests with GitHub Actions: A Fun Guide π
4 min read
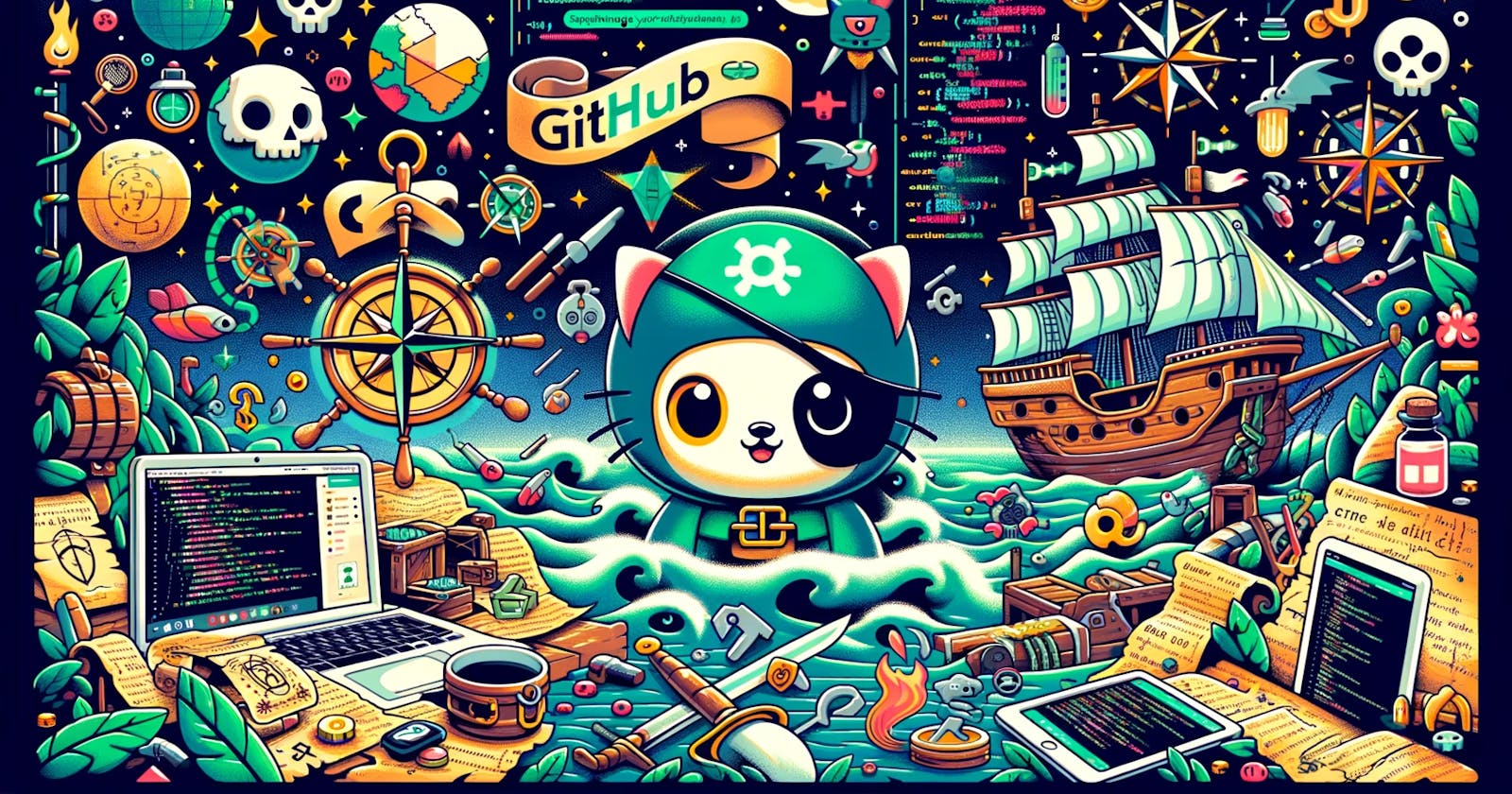
Hey there, young explorers of the coding universe! π Today, we're diving into a cool way to tell our computer programs what to do by passing them some inputs, kind of like giving directions on a treasure map! πΊοΈ In the vast sea of GitHub (our favorite spot to keep our code safe), we can use a handy tool called "GitHub Actions" to pass these directions and make our tests run exactly how we want them to.
π¬ What are Inputs?
Imagine you're the captain of a pirate ship π΄ββ οΈ, and you have a map with options: "Sail to Treasure Island ποΈ," "Explore the Mysterious Cave π΅οΈββοΈ," or "Battle the Sea Monster π." These choices are like inputs for your adventure. In GitHub Actions, we set up inputs to tell our tests things like which platform to check (Android, iOS, or Api) or what type of test to run (Smoke or Regression).
π§ Setting the Course with GitHub Actions
Our treasure map (GitHub Actions) is special because we can set it up to listen for certain signals, like when someone wants to add new code (a pull request) or when we want to start an adventure manually (workflow dispatch). Here's how we might set up our map:
name: Test Scheduler
on:
pull_request:
types:
- opened
paths:
- '**.java'
workflow_dispatch:
inputs:
platform:
description: 'Select Platform'
type: choice
options:
- Android
- iOS
- Api
required: true
default: 'iOS'
testType:
description: 'Select Test Type'
type: choice
options:
- Smoke
- Regression
required: true
default: 'Smoke'
releaseNumber:
description: 'Select release number'
type: string
required: true
This setup is like preparing your ship π’ for different adventures based on the choices made by your crew (the inputs).
π£οΈ Telling Our Code to Listen
Now, we need to make sure our code is ready to listen to these inputs. We're going to use a spell (a command) in our GitHub Actions workflow to pass these inputs to our code:
- name: Run Maven
run: mvn -Dtest=testingMain test -Dplatform=${{ inputs.platform }} -DtestType=${{ inputs.testType }} -DreleaseNumber=${{ inputs.releaseNumber }}
This command is like telling your ship's crew to get ready for an adventure based on the map's directions.
π― Inside Our Code: The Listening Part
In our Java code, we need to be prepared to use these directions:
Here is super dummy example.
import org.testng.annotations.Test;
public class testingMain {
@Test
public void testing(){
System.out.println("π------------ PLATFORM ---------------π");
String platform = System.getProperty("platform");
System.out.println("π------------ PLATFORM = " + platform + " π");
System.out.println("π------------ TEST TYPE ---------------π");
String testType = System.getProperty("testType");
System.out.println("π------------ TEST TYPE = " + testType + " π");
System.out.println("π¦------------ RELEASE NUMBER ---------------π¦");
String releaseNumber = System.getProperty("releaseNumber");
System.out.println("π¦------------ RELEASE NUMBER = " + releaseNumber + " π¦");
}
}
Our program is now all ears, ready to take action based on the adventure chosen (the inputs it received).
π Why This is Super Cool
By passing inputs like this, we can make our tests super flexible and smart! We can test on different platforms, run different types of tests, and even specify which version of our app we're testing, all without changing our code! It's like having a magical map that always knows where to find treasure. πΊοΈβ¨
π Ready for Adventure!
And with that, we're all set to sail on our coding adventures! Remember, with GitHub Actions and a bit of creativity, your code can go on all sorts of exciting journeys. So keep exploring, keep coding, and let your imagination be your guide. Happy coding, young adventurers! π’πΎπ
π Supercharge Your GitHub Actions with These Cool Ideas:
Now that you're ready to embark on your GitHub Actions adventure, here are some extra treasures to explore in your quest for coding excellence:
π Environment Selection: Choose
Development
,Staging
, orProduction
for precise environment testing.ποΈ Feature Toggle: Easily turn features
On
orOff
to test them individually.π Dynamic Test Suites: Select specific
Test Suites
orCases
to zoom in on your testing focus.π Custom Configuration Files: Swap in different
Config Files
for various testing scenarios.π Version Testing: Ensure compatibility by testing against multiple
Versions
of dependencies.π₯ User Role Simulation: Test your app's access controls by simulating different
User Roles
.π± Data Seeding Options: Pick from
Small
,Medium
,Large
, orEdgeCases
data sets for comprehensive data testing.π Internationalization Testing: Ensure your app is world-ready by testing across multiple
Locales
.
Dive into these ideas to make your GitHub Actions more dynamic and your code more robust. The world of coding is vast and full of mysteries waiting to be unraveled. Happy exploring! πππ©βπ»π¨βπ»
Yaml file example:
name: Advanced Test Workflow
on:
workflow_dispatch:
inputs:
environment:
description: 'Select Environment'
type: choice
options:
- Development
- Staging
- Production
required: true
default: 'Development'
featureToggle:
description: 'Enable New Feature'
type: boolean
required: false
userRole:
description: 'Select User Role for Testing'
type: choice
options:
- Admin
- Editor
- Viewer
required: true
default: 'Viewer'
testSuite:
description: 'Select Test Suite'
type: choice
options:
- All
- UserModule
- PaymentModule
- NotificationModule
required: true
default: 'All'
locale:
description: 'Select Locale for Testing'
type: choice
options:
- en-US
- fr-FR
- es-ES
- de-DE
required: true
default: 'en-US'