Java Decoded: Mastering the Art of Coupling for Smoother, More Efficient Code! ππ»π
3 min read
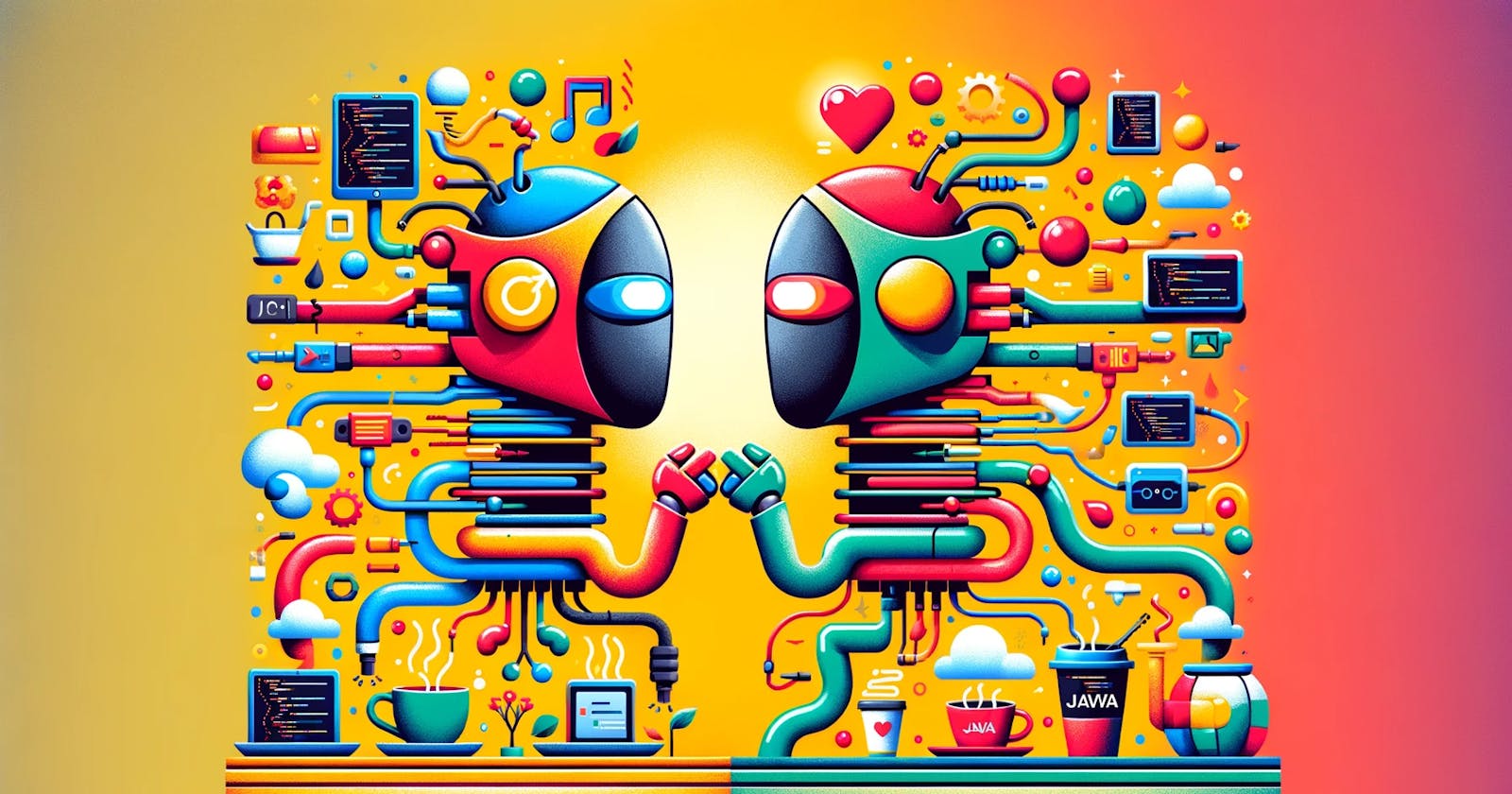
Alright, let's dive into the world of Java coupling in a fun and easy-to-understand way! π
What is Coupling in Java? π€
Coupling refers to the degree of direct knowledge that one class has about another. This isn't about the class's knowledge of another's behavior but rather its knowledge of the other class itself. Think of it as how much "chit-chat" classes have with each other. Less chit-chat (or coupling) is generally better.
Types of Coupling π§©
Tight Coupling π§²
Description: Classes are highly dependent on each other.
Example: When a class assumes too many responsibilities or when one service class uses another service class.
Pros: Easy to understand and use in small projects.
Cons: Hard to maintain, test, and extend. Changes in one class can ripple through others.
Loose Coupling π
Description: Classes are mostly independent, and changes in one class have minimal effects on others.
Example: Using interfaces or abstract classes to allow different implementations.
Pros: Easier to maintain, test, and extend. Promotes flexibility and reusability.
Cons: Can be more complex to design and implement.
When to Use? β°
Tight Coupling: In smaller, less complex projects where rapid development is needed.
Loose Coupling: In larger, scalable, and maintainable applications, especially when working with frameworks or in team environments.
Java Code Example π₯οΈ
Tight Coupling Example
class Engine {
void start() {
System.out.println("Engine started!");
}
}
class Car {
private Engine engine = new Engine();
void startCar() {
engine.start();
System.out.println("Car started!");
}
}
// Using the Car class
Car myCar = new Car();
myCar.startCar();
Loose Coupling Example
interface Engine {
void start();
}
class ElectricEngine implements Engine {
public void start() {
System.out.println("Electric Engine started!");
}
}
class Car {
private Engine engine;
Car(Engine engine) {
this.engine = engine;
}
void startCar() {
engine.start();
System.out.println("Car started!");
}
}
// Using the Car class with flexibility
Engine electricEngine = new ElectricEngine();
Car myElectricCar = new Car(electricEngine);
myElectricCar.startCar();
Pros and Cons Recap π
Tight Coupling:
Pros: Simpler to implement in small projects.
Cons: Harder to maintain, less flexible.
Loose Coupling:
Pros: More flexible, maintainable, and testable.
Cons: Can require more upfront design and planning.
Conclusion π
Understanding and applying the right type of coupling in Java is like choosing the right gear for your car. Tight coupling can be like driving in first gear - great for a quick start but not so good for the long haul. Loose coupling is like cruising on the highway, where you can smoothly change lanes and adapt to the road ahead.
Achieving loose coupling is like organizing things into categories to make them easier to use, change, and manage! ππ§Έππ»
Remember, the goal is to create a codebase that's like a well-oiled machine, easy to maintain, and a joy to expand! ππ¨ Happy coding!